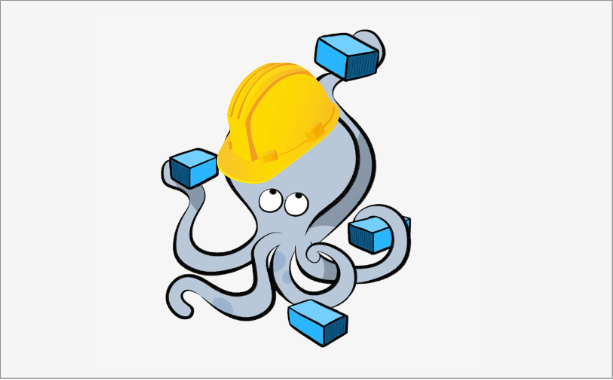
Docker Compose is a powerful tool that helps define and manage multi-container Docker applications. This article will help you master the basic commands and useful techniques when working with Docker Compose.
Basic Commands
- docker-compose up
Function: Create and start all containers defined in the docker-compose.yml file
Syntax: docker-compose up [options] [SERVICE…]
Common options:
- -d: Run in detached mode (background)
- –build: Rebuild images before starting
- –force-recreate: Recreate containers even if their configuration hasn’t changed
- –no-deps: Don’t start dependent services
Examples:
docker-compose up -d
docker-compose up -d –build app
- docker-compose down
Function: Stop and remove containers, networks, and volumes (optional)
Syntax: docker-compose down [options]
Common options:
- –volumes or -v: Remove volumes as well
- –remove-orphans: Remove containers not defined in the configuration
- –rmi [all|local]: Remove images
Examples:
docker-compose down
docker-compose down -v
- docker-compose ps
Function: List containers belonging to the project
Syntax: docker-compose ps [options] [SERVICE…]
Common options:
- -q: Only display IDs
- –services: Only display service names
Examples:
docker-compose ps
docker-compose ps -q
- docker-compose logs
Function: View logs from services
Syntax: docker-compose logs [options] [SERVICE…]
Common options:
- -f: Follow log output
- –tail=N: Display the last N lines
- –timestamps: Show timestamps
Examples:
docker-compose logs -f
docker-compose logs –tail=100 app
Container Management Commands
- docker-compose exec
Function: Execute a command in a running container (similar to SSH)
Syntax: docker-compose exec [options] SERVICE COMMAND
Common options:
- -T: Don’t allocate a pseudo-TTY
- –index=N: Specify the container if there are multiple instances
- -u USER: Run the command as a specific user
Examples:
docker-compose exec app bash
docker-compose exec -u root db mysql -u root -p
- docker-compose run
Function: Run a one-time command on a service, creating a new container
Syntax: docker-compose run [options] SERVICE [COMMAND]
Common options:
- –rm: Automatically remove the container when finished
- -d: Run in detached mode
- –no-deps: Don’t start dependent services
- -e KEY=VAL: Set environment variables
Examples:
docker-compose run –rm app npm test
docker-compose run -e NODE_ENV=test app node script.js
- docker-compose restart
Function: Restart services
Syntax: docker-compose restart [options] [SERVICE…]
Common options:
- -t, –timeout TIMEOUT: Time to wait before forcing stop (default: 10 seconds)
Examples:
docker-compose restart
docker-compose restart -t 30 app
- docker-compose stop
Function: Stop running services
Syntax: docker-compose stop [options] [SERVICE…]
Common options:
- -t, –timeout TIMEOUT: Time to wait before forcing stop (default: 10 seconds)
Examples:
docker-compose stop
docker-compose stop app db
- docker-compose start
Function: Start previously created services
Syntax: docker-compose start [SERVICE…]
Examples:
docker-compose start
docker-compose start app db
- docker-compose pause/unpause
Function: Pause or unpause services
Syntax:
- docker-compose pause [SERVICE…]
- docker-compose unpause [SERVICE…]
Examples:
docker-compose pause app
docker-compose unpause app
- docker-compose top
Function: Display running processes in each container
Syntax: docker-compose top [SERVICE…]
Examples:
docker-compose top
docker-compose top app
Container Interaction Techniques (Similar to SSH)
Docker Compose provides multiple ways to interact with containers, similar to using SSH:
- Create an interactive shell
docker-compose exec web /bin/bash
docker-compose exec app /bin/sh
- Copy files between the host machine and the container
docker cp $(docker-compose ps -q app):/app/data.json ./local/path/
- Execute commands with root privileges
docker-compose exec -u root app bash
- Execute complex shell commands
docker-compose exec app sh -c “cd /app && bash”
- Check environment variables in a container
docker-compose exec app env
- Run commands as a specific user
docker-compose exec -u postgres db psql -U postgres
- Run commands in a specific directory
docker-compose exec app sh -c “cd /var/log && ls -la”
- Transfer files from the host machine to a container
docker cp ./config.json $(docker-compose ps -q app):/app/config.json
Tips and Best Practices
- Use .env files: Store environment variables in .env files to increase security and flexibility
- Name your project: Use -p to name your project to avoid conflicts
- Use version control: Always keep your docker-compose.yml file in a version control system
- Separate development and production environments: Use separate docker-compose files for different environments
Conclusion
Docker Compose is an essential tool for managing multi-container Docker applications. With the commands and techniques presented above, you can easily deploy, manage, and debug complex applications in containerized environments.
Leave a Reply