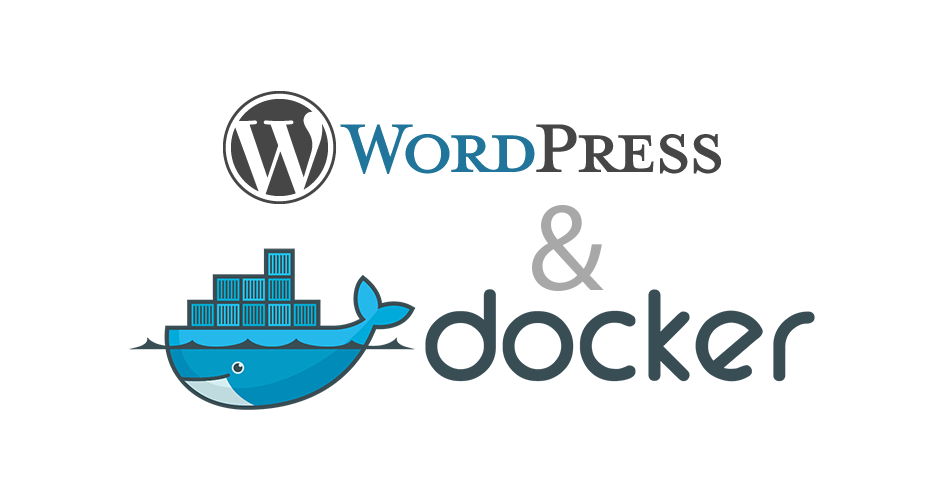
Overview of steps to build Docker for WordPress projects using Nginx as a Reverse Proxy
Main process
- Create Docker MySQL
- Set up local environment
- Set up GitLab
- Set up Ubuntu Server
- Build Docker locally and push to GitLab
- Pull WordPress source code
- Pull image from GitLab and run container
- Creating Docker for MySQL Database
Access the DB project directory on Ubuntu Server:
cd /ctech/mysql5
Create a docker-compose.yml file with the following content:
version: ‘3’
services:
db:
image: mysql:5.7.44
container_name: ctech_mysql
restart: always
ports:
– “3306:3306”
environment:
MYSQL_ROOT_PASSWORD: your_root_password
MYSQL_DATABASE: your_db
MYSQL_USER: your_user
MYSQL_PASSWORD: your_password
# Disable strict mode
MYSQL_SQL_MODE: “NO_ENGINE_SUBSTITUTION”
# Allow zero dates
MYSQL_INIT_COMMAND: “SET GLOBAL sql_mode=(SELECT REPLACE(@@sql_mode,’NO_ZERO_IN_DATE,NO_ZERO_DATE’,”));”
volumes:
– /ctech/mysql5/data:/var/lib/mysql
command: –sql-mode=”NO_ENGINE_SUBSTITUTION” –explicit_defaults_for_timestamp=1
networks:
– ctech_mysql
networks:
ctech_mysql:
name: ctech_mysql
Build Docker to pull default Docker images:
docker-compose build
Run Docker:
docker-compose up
Open port 3306 to allow remote data connections (close after completion)
Use Navicat Tools to connect via SSH Tunnel for creating databases and importing data
- Setting Up Local Environment (Windows)
Download Docker Desktop Installer.exe (choose the version compatible with your computer’s chip)
Install Docker for Windows (system restart required)
Start Docker and auxiliary services like memcache, redis (if any)
In the project directory (example: E:\xampp\htdocs\vutechsmart), create a Dockerfile:
FROM php:7.4-fpm
# Install required dependencies and PHP extensions
RUN apt-get update && apt-get install -y \
libpng-dev \
libonig-dev \
libxml2-dev \
zip \
unzip \
git \
curl
# Install PHP extensions
RUN docker-php-ext-install pdo_mysql mbstring exif pcntl bcmath gd mysqli soap
# Configure PHP as needed
RUN echo “upload_max_filesize = 64M” >> /usr/local/etc/php/conf.d/docker-php-uploads.ini \
&& echo “post_max_size = 64M” >> /usr/local/etc/php/conf.d/docker-php-uploads.ini \
&& echo “memory_limit = 256M” >> /usr/local/etc/php/conf.d/docker-php-memlimit.ini
# Set working directory
WORKDIR /var/www/html
# Ensure proper access rights
RUN chown -R www-data:www-data /var/www/html
# Expose port 9000
EXPOSE 9000
CMD [“php-fpm”]
Create a docker-compose.yml file in the project directory:
version: ‘3’
services:
wordpress:
image: ${CI_REGISTRY_IMAGE:-anhvucnt/vout-wp-php7x}:${CI_COMMIT_SHORT_SHA:-latest}
container_name: vutechsmart_wordpress
restart: always
volumes:
– /ctech/vutechsmart.com/wordpress:/var/www/html
environment:
– WORDPRESS_DB_HOST=ctech_mysql
– WORDPRESS_DB_USER=your_user
– WORDPRESS_DB_PASSWORD=your_pass
– WORDPRESS_DB_NAME=your_db
networks:
– ctech_mysql
ports:
– “9000:9000”
networks:
ctech_mysql:
external: true
name: ctech_mysql
Create a .gitlab-ci.yml file in the project directory:
stages:
– build
– deploy
variables:
DOCKER_DRIVER: overlay2
DOCKER_TLS_CERTDIR: “”
build:
stage: build
image: docker:20.10.16
services:
– docker:20.10.16-dind
before_script:
– docker login -u $CI_REGISTRY_USER -p $CI_REGISTRY_PASSWORD $CI_REGISTRY
script:
– docker build -t $CI_REGISTRY_IMAGE:$CI_COMMIT_SHORT_SHA -t $CI_REGISTRY_IMAGE:latest .
– docker push $CI_REGISTRY_IMAGE:$CI_COMMIT_SHORT_SHA
– docker push $CI_REGISTRY_IMAGE:latest
only:
– main
deploy:
stage: deploy
image: alpine:latest
before_script:
– apk add –no-cache openssh-client
– mkdir -p ~/.ssh
– echo “$SSH_PRIVATE_KEY” > ~/.ssh/id_rsa
– chmod 600 ~/.ssh/id_rsa
– echo -e “Host *\n\tStrictHostKeyChecking no\n\n” > ~/.ssh/config
script:
– ssh root@YOUR_SERVER_IP “cd /ctech/vutechsmart.com && \
docker login -u $CI_REGISTRY_USER -p $CI_REGISTRY_PASSWORD $CI_REGISTRY && \
docker pull $CI_REGISTRY_IMAGE:$CI_COMMIT_SHORT_SHA && \
docker-compose down && \
docker-compose up -d”
only:
– main
III. Setting Up GitLab
Register a GitLab account
Create SSH Key
Import SSH Key to GitLab
Create a new project on GitLab
Set up environment variables (CI/CD variables):
- SSH_PRIVATE_KEY: SSH private key to connect to the Ubuntu server
- SERVER_USER: SSH username on the server
- SERVER_IP: Server IP address
Push the project to GitLab:
git clone <repository-url>
git add .
git commit -m “Initial commit”
git push
- Setting Up Ubuntu Server
Install NGINX on Ubuntu
Access NGINX configuration:
cd /etc/nginx/sites-available
Create the configuration file vutechsmart.com:
server {
listen 80;
server_name vutechsmart.com www.vutechsmart.com;
location / {
return 301 https://$host$request_uri;
}
}
server {
listen 443 ssl;
server_name vutechsmart.com www.vutechsmart.com;
# SSL configuration
ssl_certificate /etc/letsencrypt/live/vutechsmart.com/fullchain.pem;
ssl_certificate_key /etc/letsencrypt/live/vutechsmart.com/privkey.pem;
root /ctech/vutechsmart.com/wordpress;
index index.php;
access_log /ctech/vutechsmart.com/logs/access.log;
error_log /ctech/vutechsmart.com/logs/error.log;
location / {
try_files $uri $uri/ /index.php?$args;
}
location ~ \.php$ {
fastcgi_pass 192.168.16.3:9000;
fastcgi_param SCRIPT_FILENAME /var/www/html$fastcgi_script_name;
fastcgi_index index.php;
include fastcgi_params;
}
location ~* \.(js|css|png|jpg|jpeg|gif|ico)$ {
expires max;
log_not_found off;
}
}
Important Note:
- fastcgi_pass 192.168.16.3:9000; – This IP must point to PHP-FPM in the Docker container. Check with docker network inspect ctech_mysql
- fastcgi_param SCRIPT_FILENAME /var/www/html$fastcgi_script_name; – This is the path inside the container, not on the host
Create a symbolic link to sites-enabled:
ln /etc/nginx/sites-available/vutechsmart.com /etc/nginx/sites-enabled/
Create logs directory:
mkdir -p /ctech/vutechsmart.com/logs
cd /ctech/vutechsmart.com/logs
touch access.log error.log
cd ..
chmod 0777 -R logs
Create a docker-compose.yml file on the server:
version: ‘3’
services:
wordpress:
image: registry.gitlab.com/anhvucnt/vout-wp-php7x:latest
container_name: vutechsmart_wordpress
restart: always
volumes:
– /ctech/vutechsmart.com/wordpress:/var/www/html
environment:
– WORDPRESS_DB_HOST=your_db_host
– WORDPRESS_DB_USER=your_user_host
– WORDPRESS_DB_PASSWORD=your_db_pass
– WORDPRESS_DB_NAME=your_db_name
networks:
– ctech_mysql
ports:
– “9000:9000”
networks:
ctech_mysql:
external: true
- Building Docker Locally and Pushing to GitLab
On the local environment, access the project directory:
cd E:\xampp\htdocs\vutechsmart
Log in to GitLab Registry:
docker login registry.gitlab.com -u your_gitlab_username -p your_password_or_access_token
Build Docker for testing:
docker build -t vutechsmart:test .
If there are no errors, build the official Docker:
docker build –no-cache -t registry.gitlab.com/anhvucnt/vout-wp-php7x:latest -f Dockerfile .
Push Docker image to GitLab:
docker push registry.gitlab.com/anhvucnt/vout-wp-php7x:latest
- Pulling Code and Docker Image to Ubuntu Server
Access the project directory on the server:
cd /ctech/vutechsmart.com/
Log in to GitLab Registry:
docker login registry.gitlab.com -u your_gitlab_username -p your_password_or_access_token
Pull Docker image:
docker pull registry.gitlab.com/anhvucnt/vout-wp-php7x:latest
Pull WordPress source code to /ctech/vutechsmart.com/wordpress directory
Start Docker container:
docker-compose up -d
Access your website (vutechsmart.com) to check the result
Important Note: In the Nginx configuration, pay special attention to fastcgi_pass 192.168.16.3:9000 and fastcgi_param SCRIPT_FILENAME /var/www/html$fastcgi_script_name. The IP must be the IP of the Docker container network and the path must match exactly with the path inside the container.
Good luck! 😊
Leave a Reply